How to add a custom 3rd party item to the automatic cookie blocking list
Published: 29-05-2021updated: 12-10-2023
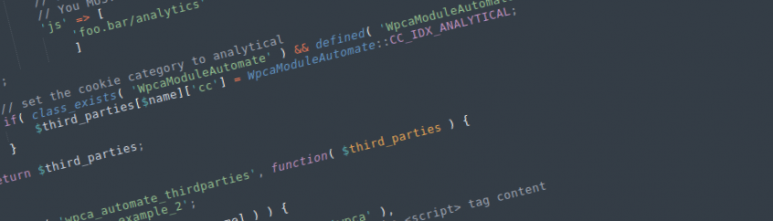
To add a custom 3rd party to the automatic cookie blocking list, you need to setup a PHP code. But first, before diving into the code directly, let’s walk through some background information:
Selectors
To block a (3rd party) script, iFrame or any other HTML element, there are 4 selectors you can use to match the 3rd party’s content:
- s = iFrame tag (e.g.
<iframe src="EXTERNAL_URL">
) - js = JavaScript script tag with source (e.g.
<script src="URL_TO_JS_SOURCE" />
) - js_needle = inline JavaScript tag (e.g.
<script>var = 'foo';</script>
) - html_elem = any HTML element (e.g.
<div>
,<section>
,<img src="EXTERNAL_URL" />
)
You can even use multiple items per selector (as an example, see example 5 about matching 2 different HTML elements with different CSS id
attribute values).
How to match
When you define the selectors, you will acctualy need to define a pattern, so that our plugin can ‘find’ (match) the HTML content and block it.
HTML elements
In the case of HTML elements (excluding <script>
tags), you’ll need to define an attribute and value as pattern.
Note that the attribute matching pattern needs to start at the beginning of the attribute value (after the first
"
), but it’s not required to define it to the end (ending"
).
When defining the element attribute name and value, always keep in mind that there can be more HTML elements with the same attribute name and (partly shared) value combination, you might not want to block. Therefore make it as unique as possible!
For example, consider the following HTML element we want to block and show a placeholder there instead:
<div id="foo-bar"></div>
The attribute name is id
and the attribute value is foo-bar
. However, defining the attribute pattern as foo-b
, foo-
or foo
would also work.
Just keep in mind that your pattern should be unique enough, so that it won’t match other <div>
elements with an id
starting with foo-b
, foo-
or foo
you not want to block.
But what if we have multiple complex and/or dynamic attribute values on one page, e.g. multiple <div>
elements with random charaters prefixed and/or suffixed:
<div class="foo-bar-fyfyfjfye"></div>
<div class="foo-bar-bkjhuhksd"></div>
or
<div class="fyfyfjfye-foo-bar"></div>
<div class="bkjhuhksd-foo-bar"></div>
In this case you can define a regular expression prefix and/or suffix [a-z]+
in your html_elem
selector (see example 7 below).
Script tags with source
In the case of a <script src="">
tag, you’ll need to define the pattern as the URL that’s inside the src
attribute.
For example, when having the following script we want to block:
<script src="https://www.foo.bar/baz.js"></script>
The src
attribute value is https://www.foo.bar/baz.js
, but because we need to omit the scheme (https://) and sub domain (www), we need to define the pattern as foo.bar/baz.js
. However, when you’re sure enough that this script URL is unique (e.g. there will be no URL like https://www.foo.bar/beer.js), you can simplify the pattern to foo.bar
or even foo
.
Inline script tags
When it comes to blocking inline script tags, you’ll need to search for a pattern which is unique for the script. Consider the following script with inline content:
<script type="text/javascript">
(function(w,p,i,e) {
console.log(w,p,i,e);
})('w','ee','p','ie')
</script>
We could set the matching pattern to function
or console
, however these are default to the JavaScript language and therefore not unique. In this case, choosing w,p,i,e
or w','ee
would be much safer.
PHP code setup
Now that we have introduced how to match your 3rd party HTML, let’s move over to setting up the PHP code.
Cookie categories
There are 5 categories defined as PHP class
constants. If you don’t set a category, it will be automatically set to other
:
- functional (
WpcaModuleAutomate::CC_IDX_FUNCTIONAL
) - analytical (
WpcaModuleAutomate::CC_IDX_ANALYTICAL
) - social media (
WpcaModuleAutomate::CC_IDX_SOCIAL_MEDIA
) - advertising (
WpcaModuleAutomate::CC_IDX_ADVERTISING
) - other (
WpcaModuleAutomate::CC_IDX_OTHER
)
The basics
The PHP code is a call to WordPress’s add_filter()
function with a callback function:
add_filter( 'wpca_automate_thirdparties', function() {
} );
where wpca_automate_thirdparties
is a WordPress hook our plugin uses to filter the 3rd party list. For the 3rd party to be added, inside the callback we will define:
- a unique internal name
- patterns which are needed to match our 3rd party
So in the callback, start with the internal name definition:
// Give your 3rd party an internal name
// Only use lowecase letters, numbers, underscores, hyphens
$name = 'foobar';
Then, define an array
inside the if
statement, that defines the label and (1 or more) selectors with patterns:
if( !isset( $third_parties[$name] ) ) {
$third_parties[$name] = [
// The label that will show up at the General settings 3rd party overview
'label' => __( 'Foo Bar 3rd party', 'wpca' ),
// If you want to match an iFrame tag with `src` attribute
's' => [
'UNIQUE_PATTERN'
],
// If you want to match a JavaScript tag with `src` attribute
'js' => [
'UNIQUE_PATTERN'
],
// If you want to match an inline JavaScript tag
'js_needle' => [
'UNIQUE_PATTERN'
],
// If you want to show a placeholder or just block an (pixel) image
'html_elem' => [
'name' => 'HTML_ELEMENT_NAME',
'attr' => 'ATTRIBUTE_NAME:UNIQUE_PATTERN'
]
];
}
How to apply the custom 3rd party
- Copy paste your PHP code (see examples below) into your Theme’s functions.php file:
- Click the “Refresh” button at the “General” settings page:
- Check the newly created entry in the thirdparty items table:
- Click the “Save changes” button:
Examples
The following examples illustrate most common use cases:
example 1: match a script with src attribute
<script src="https://www.foo.bar/analytics.js"></script>
add_filter( 'wpca_automate_thirdparties', function( $third_parties ) {
$name = 'foobar_example_1';
if( !isset( $third_parties[$name] ) ) {
$third_parties[$name] = [
'label' => __( 'Foo Bar example 1', 'wpca' ),
// Note: you only need to give a fragment of the whole URL,
// ommiting https://www. and the last part (/ad.js).
// You MUST begin after the scheme and/or sub domain!
'js' => [
'foo.bar/analytics'
]
];
// set the cookie category to analytical
if( class_exists( 'WpcaModuleAutomate' ) && defined( 'WpcaModuleAutomate::CC_IDX_ANALYTICAL' ) ) {
$third_parties[$name]['cc'] = WpcaModuleAutomate::CC_IDX_ANALYTICAL;
}
}
return $third_parties;
} );
example 2: match a script with inline javascript code
<script type="text/javascript">
(function(d,s,e,t){e=d.createElement(s);e.type='text/java'+s;e.async='async';
e.src='http'+('https:'===location.protocol?'s://s':'://')+'www.foo.bar/ad.js';
t=d.getElementsByTagName(s)[0];t.parentNode.insertBefore(e,t);})(document,'script');
</script>
add_filter( 'wpca_automate_thirdparties', function( $third_parties ) {
$name = 'foobar_example_2';
if( !isset( $third_parties[$name] ) ) {
$third_parties[$name] = [
'label' => __( 'Foo Bar example 2', 'wpca' ),
// Pass a pattern (needle) that occurs in the <script> tag content
// (as unique as possible!)
'js_needle' => [
'foo.bar/ad.js'
]
];
// Set the cookie category to advertising
if( class_exists( 'WpcaModuleAutomate' ) && defined( 'WpcaModuleAutomate::CC_IDX_ADVERTISING' ) ) {
$third_parties[$name]['cc'] = WpcaModuleAutomate::CC_IDX_ADVERTISING;
}
}
return $third_parties;
} );
example 3: match an iFrame
<iframe src="https://www.foo.bar/ad.html"></iframe>
add_filter( 'wpca_automate_thirdparties', function( $third_parties ) {
$name = 'foobar_example_3';
if( !isset( $third_parties[$name] ) ) {
$third_parties[$name] = [
'label' => __( 'Foo Bar example 3', 'wpca' ),
// Note: you only need to give a fragment of the whole URL,
// ommiting https://www. and the last part (/ad.html).
's' => [
'foo.bar'
]
];
// Set the cookie category to advertising
if( class_exists( 'WpcaModuleAutomate' ) && defined( 'WpcaModuleAutomate::CC_IDX_ADVERTISING' ) ) {
$third_parties[$name]['cc'] = WpcaModuleAutomate::CC_IDX_ADVERTISING;
}
}
return $third_parties;
} );
example 4: match a custom HTML element
<section id="foo-bar-section"></section>
add_filter( 'wpca_automate_thirdparties', function( $third_parties ) {
$name = 'foobar_example_4';
if( !isset( $third_parties[$name] ) ) {
$third_parties[$name] = [
'label' => __( 'Foo Bar example 4', 'wpca' ),
'html_elem' => [
// Match the element name "section" from <section>
'name' => 'section',
// Match the "id" attribute and value "foo-bar-section"
'attr' => 'id:foo-bar-section'
]
];
// set the cookie category to other
if( class_exists( 'WpcaModuleAutomate' ) && defined( 'WpcaModuleAutomate::CC_IDX_OTHER' ) ) {
$third_parties[$name]['cc'] = WpcaModuleAutomate::CC_IDX_OTHER;
}
}
return $third_parties;
} );
example 5: match multiple HTML elements
<div id="foo-bar-wine"></div>
<div id="foo-bar-beer"></div>
add_filter( 'wpca_automate_thirdparties', function( $third_parties ) {
$name = 'foobar_example_5';
if( !isset( $third_parties[$name] ) ) {
$third_parties[$name] = [
'label' => __( 'Foo Bar example 5', 'wpca' ),
// Notice that the multiple `html_elem` items
// are arrays themselfs: [ [], [] ]
'html_elem' => [
[
// Match the element name "div" from <div>
'name' => 'div',
// Match the "id" attribute and value "foo-bar-wine"
'attr' => 'id:foo-bar-wine'
],
[
// Match the element name "div" from <div>
'name' => 'div',
// Match the "id" attribute and value "foo-bar-beer"
'attr' => 'id:foo-bar-beer'
]
]
];
// set the cookie category to other
if( class_exists( 'WpcaModuleAutomate' ) && defined( 'WpcaModuleAutomate::CC_IDX_OTHER' ) ) {
$third_parties[$name]['cc'] = WpcaModuleAutomate::CC_IDX_OTHER;
}
}
return $third_parties;
} );
example 6: a combination of multiple selectors
<div class="foo-bar"></div>
<script type="text/javascript">
function fooBarFn() {}
</script>
<script src="https://www.foo.bar/ad.js"></script>
add_filter( 'wpca_automate_thirdparties', function( $third_parties ) {
$name = 'foobar_example_6';
if( !isset( $third_parties[$name] ) ) {
$third_parties[$name] = [
'label' => __( 'Foo Bar example 6', 'wpca' ),
// Match the inline script with the pattern "fooBarFn("
'js_needle' => [
'fooBarFn('
],
// Match the source script with the URL pattern "foo.bar"
'js' => [
'foo.bar'
],
'html_elem' => [
// Match the element name "div" from <div>
'name' => 'div',
// Match the "class" attribute and value "foo-bar"
'attr' => 'class:foo-bar'
]
];
// set the cookie category to other
if( class_exists( 'WpcaModuleAutomate' ) && defined( 'WpcaModuleAutomate::CC_IDX_OTHER' ) ) {
$third_parties[$name]['cc'] = WpcaModuleAutomate::CC_IDX_OTHER;
}
}
return $third_parties;
} );
example 7 (advanced): using a regular expression to match a dynamic attribute value
<div class="foo-bar-fyfyfjfye"></div>
<div class="foo-bar-bkjhuhksd"></div>
add_filter( 'wpca_automate_thirdparties', function( $third_parties ) {
$name = 'foobar_example_7';
if( !isset( $third_parties[$name] ) ) {
$third_parties[$name] = [
'label' => __( 'Foo Bar example 7', 'wpca' ),
'html_elem' => [
// Match the element name "div" from <div>
'name' => 'div',
// Match the "class" attribute and value "foo-bar-"
// (notice the ending "-")
'attr' => 'class:foo-bar-',
// Match 1 or more a-z characters after the (suffixed)
// `foo-bar-` value.
// When the dynamic part of an attribute is at the beginning,
// you can use a `regex_prefix` key instead.
'regex_suffix' => '[a-z]+'
]
];
// set the cookie category to other
if( class_exists( 'WpcaModuleAutomate' ) && defined( 'WpcaModuleAutomate::CC_IDX_OTHER' ) ) {
$third_parties[$name]['cc'] = WpcaModuleAutomate::CC_IDX_OTHER;
}
}
return $third_parties;
} );
example 8 (advanced): define a JavaScript (window) dependency
<script type="text/javascript">
(function(a) {
a.init();
})(myGlobalVar)
</script>
add_filter( 'wpca_automate_thirdparties', function( $third_parties ) {
$name = 'foobar_example_8';
if( !isset( $third_parties[$name] ) ) {
$third_parties[$name] = [
'label' => __( 'Foo Bar example 8', 'wpca' ),
'js_needle' => [
// Match the script specific content "a.init("
's' => 'a.init(',
// Only reload this script when the `myGlobalVar` is ready.
// Our plugin checks if it's available in the `window` object.
'dep' => 'myGlobalVar',
// Retry finding the dependency with an interval of 200ms.
'dt' => 200
]
];
// set the cookie category to other
if( class_exists( 'WpcaModuleAutomate' ) && defined( 'WpcaModuleAutomate::CC_IDX_OTHER' ) ) {
$third_parties[$name]['cc'] = WpcaModuleAutomate::CC_IDX_OTHER;
}
}
return $third_parties;
} );